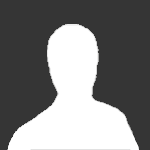
Mr. Mos In-Depth Scenes and Windows
By
Guest Mr Mo, in Archived RPG Maker XP Tutorials
-
Recently Browsing 0 members
No registered users viewing this page.
By
Guest Mr Mo, in Archived RPG Maker XP Tutorials
No registered users viewing this page.